Error: Too Much Recursion
long story short, chrome will make this easier, but chrome was broken on my machine.
Turns out sed pulled through nicely for front-end troubleshoting.
The trouble boils down to firefox. This came up for me when I forgot to comment out a call during some refactoring.
Firefox’s problem
if you want the error, run this mess of code in firefox:
larry = function(issue){ curly(issue) }
curly = function(issue){ moe(issue) }
moe = function(issue){ larry(issue) }
tom = function(issue){ moe(issue) }
dick = function(issue){ manager(issue) }
harry = function(issue){ john(issue) }
bob = function(issue){ harry(issue) }
john = function(issue){ dick(issue) }
manager = function(issue){
console.log('resolved issue')
}
bob('new issue')
moe('another new issue')
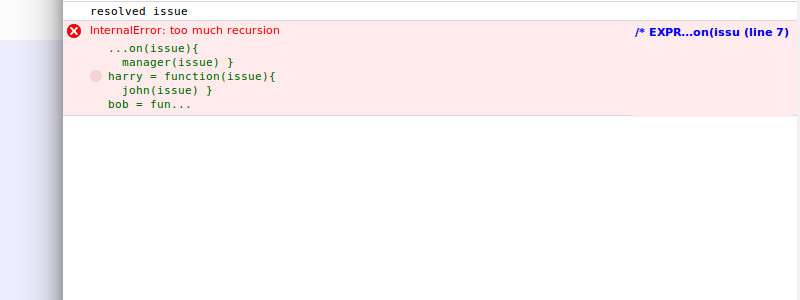
right about now you might want to start setting breakpoints, but hold tight.
you sed fix it?
use sed to make a slapdash call stack:
#!/bin/sed -si -rf
# call me with ./*.js
# scope things -- mostly for readability
/function/{
# for declarations
/^ *function/{
s/^ *function ([[:alpha:]]+) ?\(.*$/&\n\1/
}
# for expressions
/= *function/{
s/^ *(var )?([^ ]*) =.*$/&\n\2/
}
s|^([^\n]*)\n(.*)$|\1\nconsole.log('\2')// fakeCallStack|
}
or a simpler one-liner:
sed -rsi '/= *function/{ s/^ *(var )?([^ ]*) =.*$/&\nconsole.log("\2")/ }' ./*.js
and your functions start logging themselves!
larry = function(issue){
console.log('larry')// fakeCallStack
curly(issue) }
fire it up in your browser and logs will go flying by:
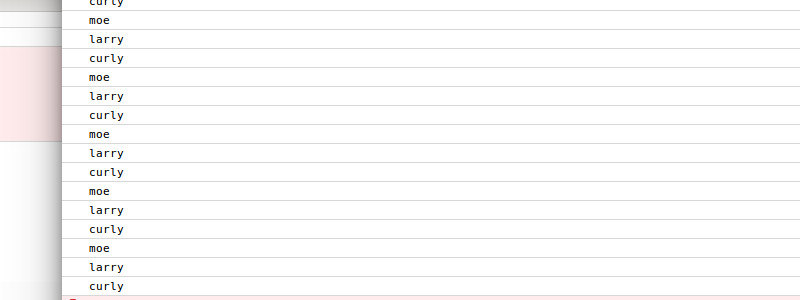
the culprits. Now you’re shooting fish in a barrel.
Search for the functions in your ide of choice or grep -Hnr '<your function name>' ./
chrome gets it right
Chrome logs the call stack for you.
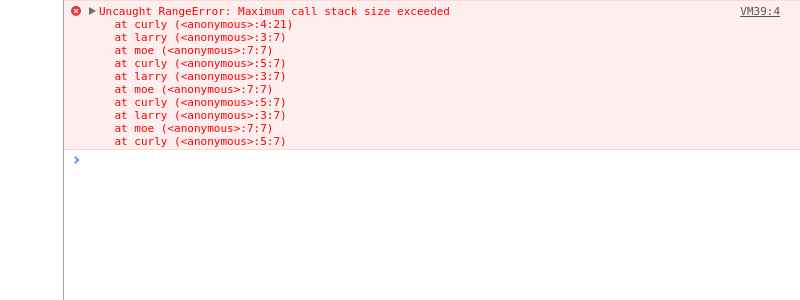
problem miraculously solved!
but not that miraculous…